Last week I talked about my experiences with teaching lists to new programmers using the Scratch programming language. In this post I’ll walk through an example, showing how lists can greatly simplify a program that has a lot of similar variables.
Let’s say your teacher has asked you to help write a program to determine the percentage of tests that received a passing grade. If a test scored above 68 points then it passed. If it scored 68 or less points then it failed. The passing percentage is the total number of tests that passed divided by the overall number of tests. For example, if there were four tests and three of them received a passing grade, then you would divide 3 by 4 to determine that 75% had passed.
In this example, your teacher has given you five test scores. Since five is an easily managed number, you decide to create five variables in your program to keep track of them. You also create a Passed variable to track the total number of passing tests. Combined with five If Statements, you can increment the Passed variable to determine the total number of passing tests. Finally, divide that variable by five (the total number of tests) to determine the percentage.
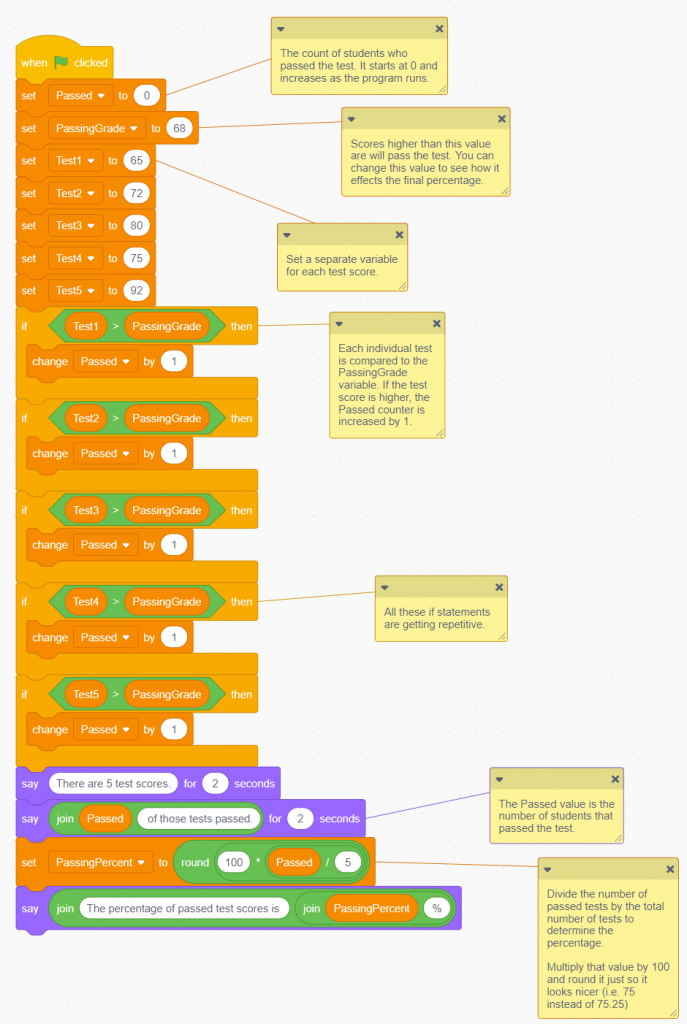
The program worked great and got the teacher the needed information. However, then the teacher surprised you and said there were actually somewhere between 8,000 and 9,000 tests. Using that many variables would be a ton of repetitive code. There would be a lot of opportunity for typos to slip in and it wouldn’t be very much fun to write. Plus, you don’t even know exactly how many variables there will be.
This is a great example of where Lists are incredibly useful. Let’s run through a quick explanation of the list blocks in Scratch.
How Lists Work in Scratch
A list is a special type of variable that can hold multiple variables within it. Lists are created from the section containing Variable blocks. When you create a new list and name it, several new blocks will appear. These blocks can be used to add to, delete from, or make other changes to a list.
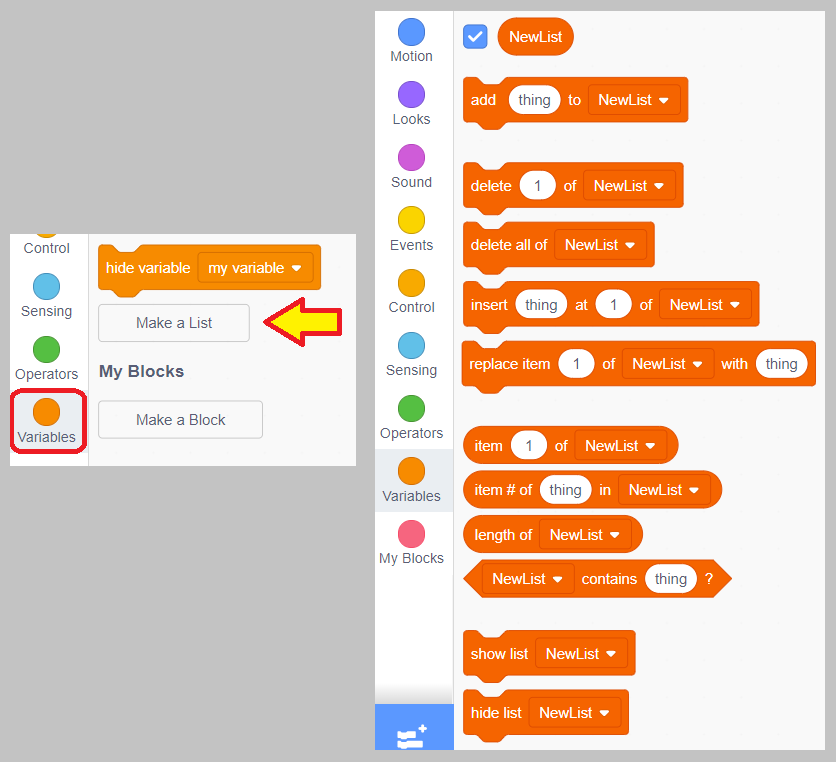
When a new list is created, a box will also appear on the screen where you can enter multiple values. Simply press the plus sign on the lower left corner to add new items.
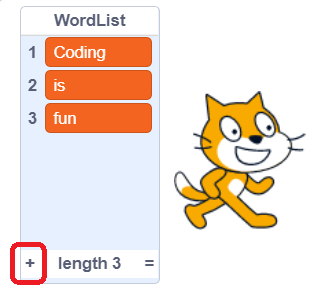
When you type items into the list, notice that a number appears on the left hand side. This number is called the index and is the key to accessing individual items from a list. One of the most common blocks is the item of list block. With this block, you can type in the index number you want and select the list from the drop down menu (assuming you have created more than one list). That block will then act like a regular variable.
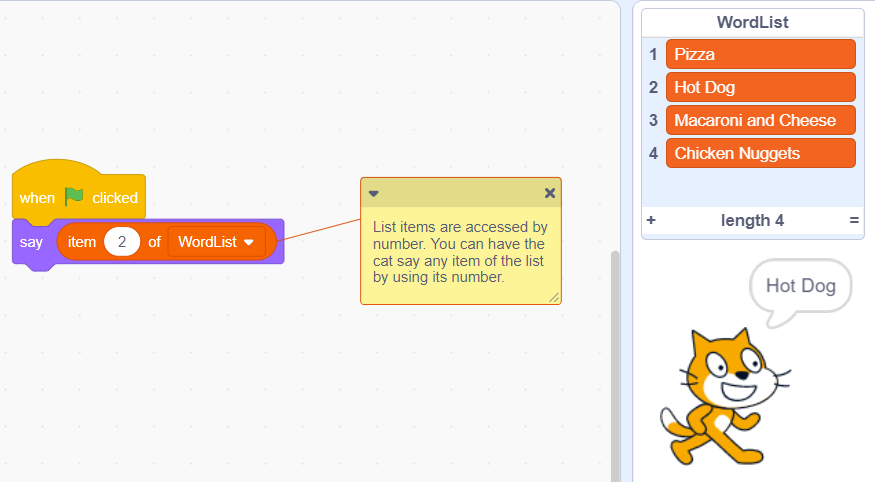
One of the most commonly used applications of lists is to apply a program to every item in the list by looping through it. For example, you could have a list of numbers and perform a math problem on each one. Or you could have a list of student names and then have a program that said “Hello NAME” to each of them. There are other more advanced applications like finding the maximum value, average value, or longest word in a list.
Regardless of why you want to access every value in a list, you will usually do so with a Repeat Block. One of the list blocks is called the Length of List block. This block’s value will always be the number of items in the list named in the drop down box and will be updated as items are added or removed from the list.
- This block can be added to a Repeat Block in the same way that a typed number or regular variable could be.
- This will run the Repeat Block as many times as there are items in the list.
- You should also create a counter variable that will be incremented by one each time the loop runs.
- You can use the counter variable as the index in the Item of List block to access each item in the list one at a time.
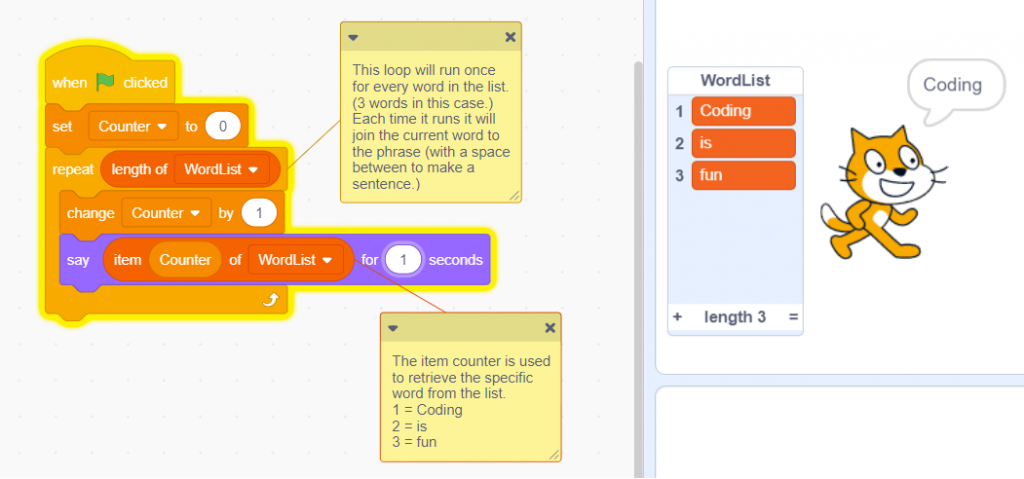
Finding the Percentage of Passing Tests with a List
With these few blocks, we can get back to helping the teacher determine the percentage of passing tests – even when there are thousands of them.
I’ve created a quick process at the start of the program to fill a random number of tests scores (between 8,000 and 9,000) with a random score between 60 and 100. The exact number of tests and the scores on the list will be different every time the program is executed. This means the percentage will be different every time the program runs.
The logic to calculate the percentage of scores is the same as in the variable example at the start of this post. But now, instead of executing the calculation five separate times, it is part of a Repeat block that is executed once for every item in the list.
- Every time the code in the Repeat Block runs it adds one to the Counter variable.
- It uses the Counter variable as the index value in the Item of List block.
- If that item’s value is higher than the PassingGrade variable, the Passed variable is increased by one.
- After the Repeat Block has run for every item in the list, the Passed Variable is divided by the length of the list to determine the percentage of passing scores.
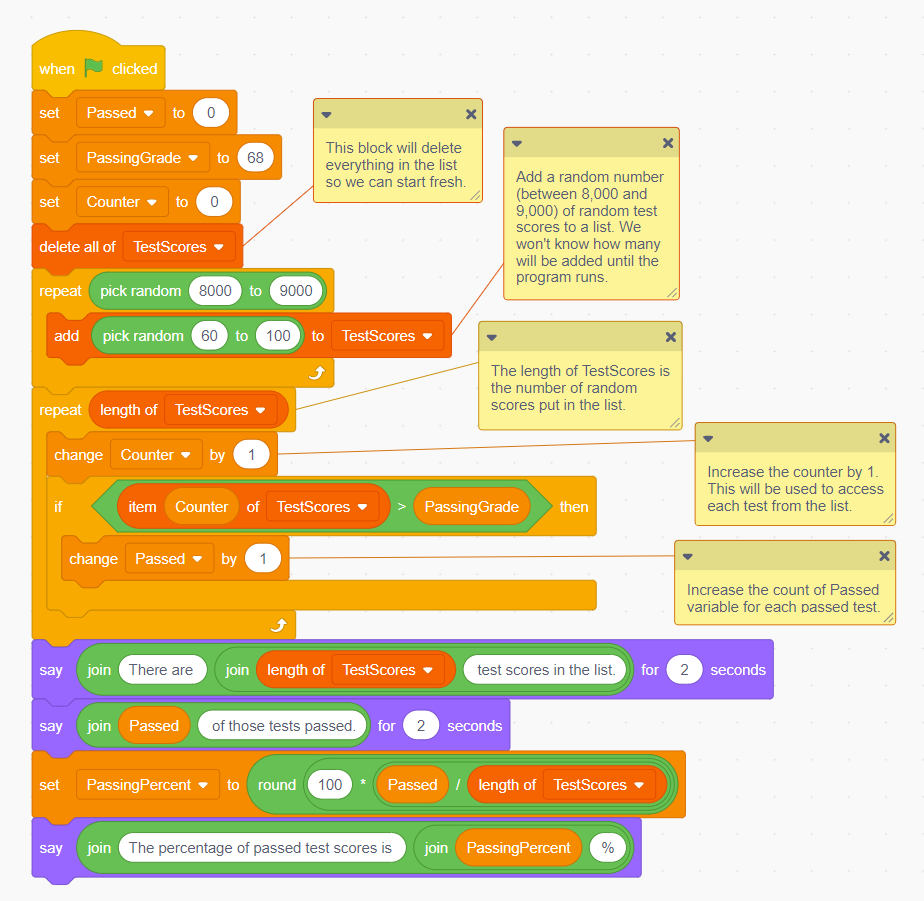
This example helps illustrate a problem that variables alone can’t solve because there is too much data. When I taught this in my daughter’s coding club, the kids seemed to grasp the concept of why they might want to use a list. The example of processing large amounts of data may not be as much fun as programming a small game, but it represents some of the challenges that professional programmers use to solve problems.
You can access all programs from this example on Scratch.
Test Score Grader with Variables
Test Score Grader with Lists
Example of the Item of List Block
Simple List with Loop (Coding is fun)